VPhysics
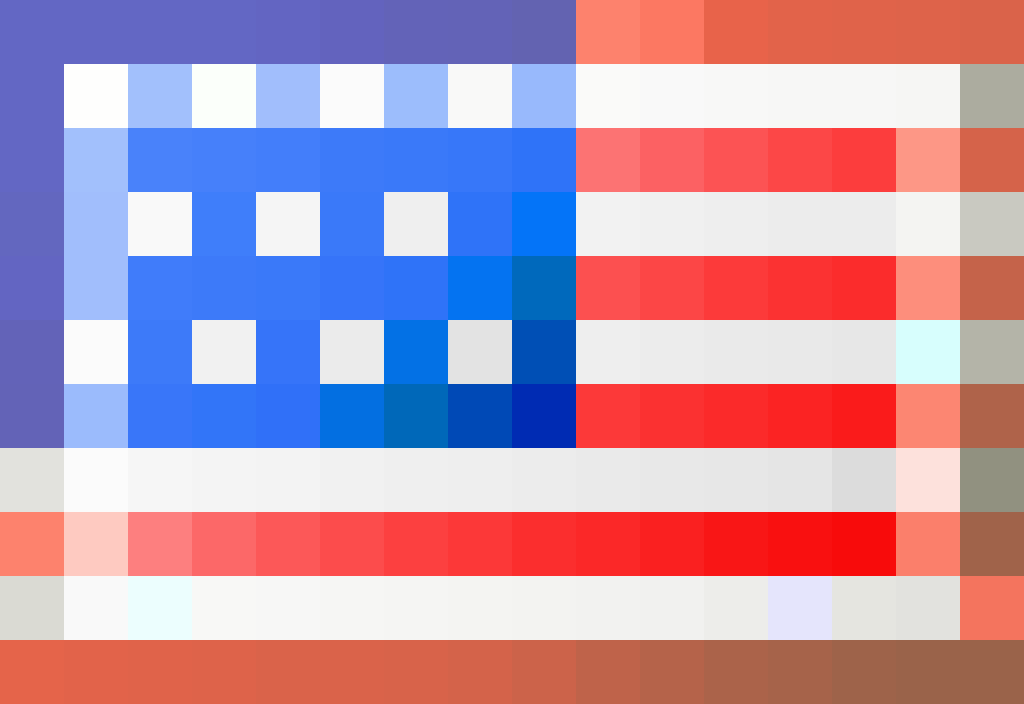

VPhysics is Source's built-in 3D physics simulation engine. VPhysics objects move and collide believably thanks to the system's simulation of mass, gravity, friction, air resistance, inertia, and buoyancy.
VPhysics was originally created by incorporating Ipion Virtual Physics, a physics middleware acquired and since licensed by Havok (later acquired by Microsoft). The successor to VPhysics on Source 2 is Rubikon.
Contents
Collision Models
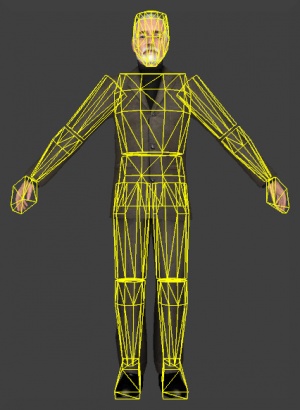
VPhysics objects ("collision models") are invisible, because in order for the simulation to be efficient they must be extremely simple. The visible part of an entity will obviously be a close match to the shape of the VPhysics object, but it is entirely ignored by the simulator.
Collision models are typically loaded from a collision mesh embedded inside an entity's assigned model, but they can also be generated, from a bounding box for instance. Spherical collision models are also possible.

vcollide_wireframe 1
.QPhysics
VPhysics co-exists with QPhysics (a retroactive name—it's only ever referred to in the codebase as "game physics"), an older and far less sophisticated simulator that Source inherits from Quake II. QPhysics is still used for players and walking NPCs because full VPhysics would lead to situations too complex for either AI or players to handle. Vehicles and flying NPCs like npc_manhack do use VPhysics, however. Most moving brush entities (func_rotating, func_movelinear, func_tracktrain etc) also use QPhysics to move.
QPhysics entities are given a VPhysics "shadow" that allows them to interact with VPhysics entities in a limited way. They can push around physics objects, and be an anchor for physics constraints, but cannot be influenced the other way around. Some brush entities can be temporarily blocked by physics objects.
Brush entities use a combination of axial and planar brushsides for QPhysics collision, and point entities use axial bounding boxes or hitboxes. Rotating QPhysics collision will cause it to subtly break, such as making surfing impossible.
Programming
In programming terms, VPhysics is an engine library that takes direct control of the motion of an entity. When it is in full effect QPhysics positioning functions cease to have any effect, and the object's behaviour is instead influenced through the application of forces and constraints (though direct modification is still possible).
This spawn function creates a physically-simulated model:
#define MODEL "models/props_c17/FurnitureDresser001a.mdl"
void CMyEnt::Spawn()
{
PrecacheModel(MODEL);
SetModel(MODEL);
VPhysicsInitNormal(SOLID_VPHYSICS,0,false);
}
There initialisation functions are:
VPhysicsInitNormal
- Used above. Makes the entity a free VPhysics object with full simulation and collisions.
VPhysicsInitStatic()
- An object that never moves.
VPhysicsInitShadow( bool allowPhysicsMovement, bool allowPhysicsRotation, solid_t *pSolid = 0 )
- An object that collides with other VPhysics objects, but has its location defined by the QPhysics motion of its entity. This can lead to very strong forces being exerted on normal VPhysics objects, so use with care!
Unlike VPhysicsInitNormal()
, neither of the functions above implicitly call SetSolid()
. You will need to do so yourself as the default SOLID_NONE
causes both to return early.

SetSolid()
alone does not enable VPhysics collisions.Units
Measurement | Unit |
---|---|
Distance | Inches |
Mass | Kilogrammes |
Volume | Cubic inches |
Velocity | Inches per second |
Acceleration | Inches per second per second |
Density | Kilogrammes per cubic metre |
Force | Inches per second per kilogramme |
Torque | Degrees per second per kilogramme |
Structures
IPhysicsEnvironment
- A VPhysics environment within which objects are simulated. New
IPhysicsObject
s are created here, and global settings like gravity can be configured. IPhysicsEnvironment* physenv
is created by default. Multiple environments are entirely possible, which is howPortal and
Portal 2 work.
IPhysicsObject
- The core interface into a VPhysics object. Provides functions for tweaking parameters and applying forces. An entity can have multiple
IPhysicsObject
s (e.g. ragdolls). IPhysicsCollision
- Provides various
CPhysCollide
andCPhysConvex
(see below) utilities. Use thephyscollision
global instance. CPhysCollide
- A rigid collision mesh. Despite being named like a server entity, this class is defined in the engine. It can be passed to various
IPhysicsCollision
functions. vcollide_t
- A collection of
CPhysCollide
s. CPhysConvex
- A single, convex collision mesh element.
CPhysPolysoup
- A collection of raw polygons that represent a collision mesh. Call
physcollision->ConvertPolysoupToCollide()
to "compile" it to aCPhysCollide
. solid_t
- A collection of data passed to the engine when creating new physics objects.