PCapture-Lib - Portal 2 VScript Library
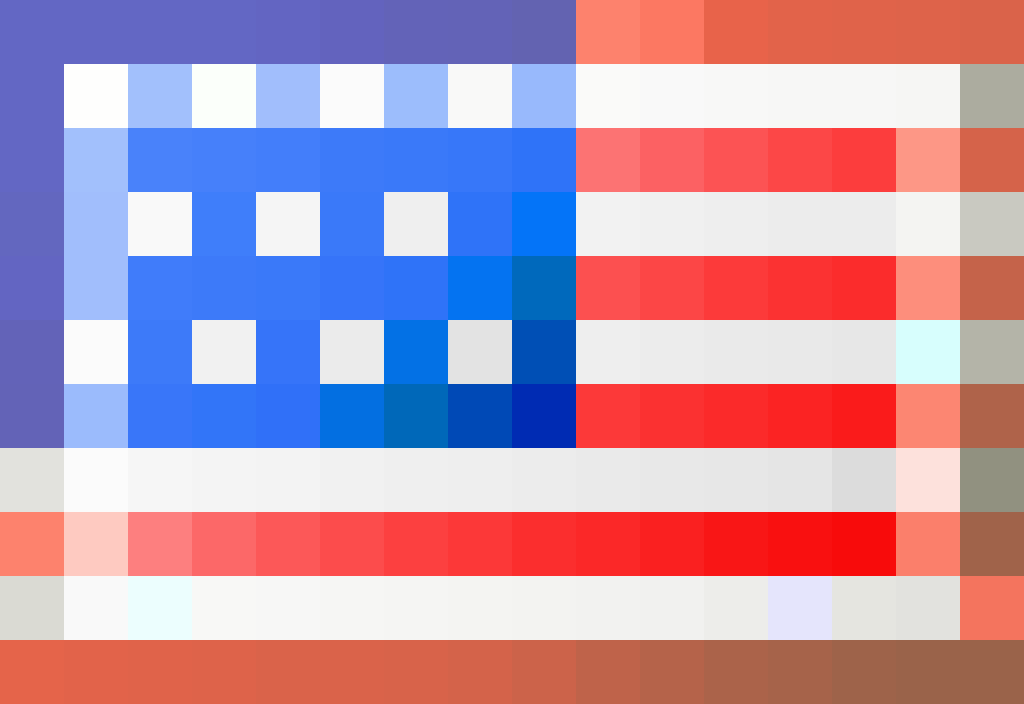


You can upload screenshots at Special:Upload. For help, see Help:Images.
Template:Pcaplib is a comprehensive and open-source VScripts library for
Portal 2, designed to significantly enhance VScript capabilities and facilitate the creation of more engaging and complex gameplay. It provides a wide range of tools to address common scripting challenges, empowering developers to realize more ambitious and immersive maps.
This page provides a basic overview of the library.
About
The library is compatible with all Source Engine games that support VScripts 2+ (in all games since
). Support for
VScripts 3+ will be added in the future.
PCapture-Lib originated from the Project Capture modification and is actively maintained by laVashik, an experienced Portal 2 modder known for their work on projects such as NullPoint Crisis, MultiPortals, and Fanctronic.
GitHub Repo: PCapture-Lib
To report bugs or problems, please create an issue on the GitHub repository.
Features
PCapture-Lib offers a wealth of features designed to boost your Portal 2 modding workflow:
Advanced Ray Tracing (TracePlus)
Go beyond basic raycasts. This module includes:
- Portal Support: Trace rays that seamlessly travel through portals.
- BBox Casting: Optimized bounding box collision detection.
- Cheap Traces: Fast, less resource-intensive traces.
- Customizable Settings: Fine-tune trace behavior.
- From Eyes Traces: Trace from the player's viewpoint.
Enhanced Data Types (IDT)
Work with improved data structures:
- ArrayEx: A powerful array extension.
- List: A doubly-linked list.
- AVLTree: A self-balancing binary search tree.
- pcapEntity: A comprehensive wrapper for `CBaseEntity`.
- entLib: Facilitates easy entity creation and searching.
Action Scheduler
Create complex timed sequences with ease:
- Scheduled Actions: Define actions that trigger after a delay.
- Interval Actions: Execute actions repeatedly at fixed intervals.
- Event Management: Cancel events by name or action.
- Asynchronous Actions: Use the `yield` keyword for time-based operations.
Animations
Animate entity properties smoothly:
- Pre-built Transitions: Alpha, color, position, and angle transitions.
- Real-Time Animations: For dynamic control.
- Customization: Fine-tune easing, duration, and more.
Script Events
Build modular and event-driven systems:
- VGameEvent: Create and manage custom game events.
- EventListener: Centralized event notification and handling.
- Simplified Logic: Decouple code with custom event triggers.
HUD Enhancements (HUD)
Create informative on-screen elements:
- ScreenText: Display customizable text on the screen.
- HintInstructor: Show hints and instructions.
Comprehensive Math Module
A wide array of mathematical tools:
- Basic Algebra: `min`, `max`, `clamp`, `round`, etc.
- Vector Utilities: Equality checks, rotation, reflection, etc.
- Linear Interpolation (lerp): Interpolate numbers, vectors, colors.
- Easing Equations: Smooth animations (InSine, OutSine, InOutCubic, etc.).
- Quaternions: Advanced rotation calculations.
- Matrices: Linear transformations.
Utilities (Utils)
- Debugging: Bounding box visualization, leveled logging.
- File Operations: Simplified file reading and writing.
- Improvements: Enhanced `FrameTime()`, `UniqueString()`, `EntFireByHandle()`.
- Macros: Shortcuts for common operations.
- Player Hooks: Simplified player access, automatic player tracking.
Installation
- Download: Download the latest release of PCapture-Lib.nut from the Releases page.
- Place: Put PCapture-Lib.nut in your mod's portal2/scripts/vscripts directory (or a subdirectory within it).
- Import: Add this line to your VScript file: IncludeScript("PCapture-Lib")
Documentation
For in-depth documentation and examples, visit the GitHub repository. Each module has its own `readme.md` file.