Sound operators
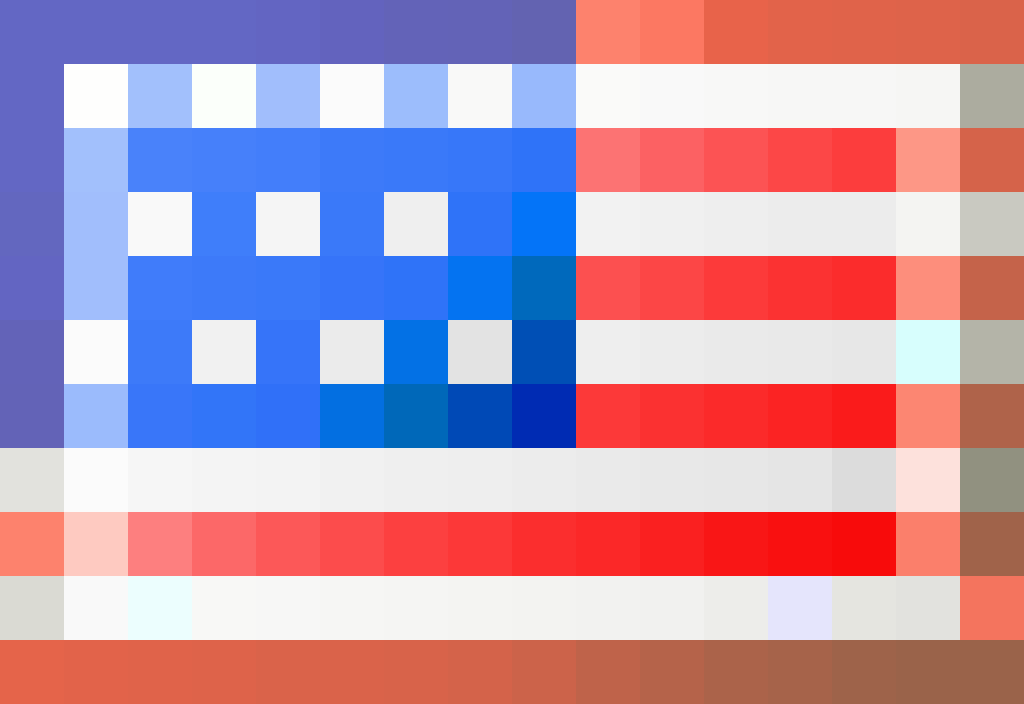

These are the root sound operators referenced in Portal 2. See scripts/sound_operator_stacks.txt
and Soundscripts#Operator stacks for more details.
To chain operators together, values can be set to @prev_op_name.property_name
to read a value. For vectors the names can be appended with an index in square brackets to set/get a specific value.
Usage
VFX.LightFlickerEnd
{
channel CHAN_AUTO
soundlevel SNDLVL_105db
volume 1.0
rndwave
{
wave "vfx/light_flicker/light_flicker_end_01.wav"
wave "vfx/light_flicker/light_flicker_end_02.wav"
wave "vfx/light_flicker/light_flicker_end_03.wav"
wave "vfx/light_flicker/light_flicker_end_04.wav"
}
soundentry_version 2
operator_stacks
{
start_stack // applied when the sound begins
{
import_stack "P2_exclusion_time_blocker_start" // defined in scripts/sound_operator_stacks.txt
// We are now extending/configuring P2_exclusion_time_blocker_start
block_entries // prevents another sound from playing
{
input_duration 0.25 // seconds to block for
match_entry "World.LightFlickerEnd" // the sound entry to block
match_entity false // only on the same entity that this sound is playing from?
}
}
}
}
Common properties
execute_once
- Whether the operator should run every time the stack is evaluated, or only the first time. Output values are presumable stored between executions in the latter case.
input_execute
- The operator will only run if this value is true (i.e. non-zero).
output
- The result of the operator (if there is one). Accessed with
@OperatorName.output
.
There is also one special command that is not an operator:
import_stack
- Inserts another named stack into this one.
Operator list
System
Affect sound playback.
sys_stop_entries
Finds currently playing sounds and stops the matching ones, optionally with a delay. Its essential properties are match_entry
and/or match_sound
to specify one or more sounds to stop.
Input Name | Type | Default | Description |
---|---|---|---|
input_execute |
float | 1.0 | If set to a value smaller or equal to 0.0 , no stopping is performed.
|
input_max_entries |
float | 1.0 | If set to x , stops as many matching sounds until there are less than x of them still playing. This usually does not include the new instance of the sound entry containing this operator: When used in a start_stack to stop instances of itself, setting this to n allows up to n+1 instances playing simultaneously.
![]() |
input_stop_delay |
float | 0.0 | If some instance of a sound entry will be stopped, then with this delay in seconds. |
match_entry |
string | "" | If this is not the empty string, matches only sounds that originate from a sound entry with this exact name (not case sensitive). If match_substring is set to true, matches all sounds whose sound entry name contains the specified string.
|
match_sound |
string | "" | If this is not the empty string, matches only sounds where the string of the path of their audio file equals the specified string (not case sensitive). For currently playing sounds, it's the path shown at the top right of the screen when snd_show 1 is set, which means that the string includes preceding sound characters as well as backslashes \ between directories (not forward slashes / ).If the property match_substring is true, all entries will be matched that contain this string in their path string.
|
match_substring |
bool | false | Alters the matches for both match_entry and match_sound . If set to false, those strings must be exactly equal. For example, if set to true, MyGun matches both sound entries MyGun.fire and MyGun.reload (because both entries contain the sequence MyGun ).
|
match_channel |
bool | false | If set to true, sounds are only matched if they are in the same channel (e. g. CHAN_STATIC ) as this sound entry.
|
match_entity |
bool | false | If set to true, sound are only matched if they are coming from the same entity as this sound entry. |
stop_this_entry |
bool | false | Whether this entry (the one containing the operator stack where this property appears) should be stopped. If true, this property is sufficient to target a sound to stop. This property appears only to be usable in an update_stack , probably because when the code for (pre)start_stack is executed, the sound itself doesn't yet exist and thus can't reference itself at that moment.
|
match_this_entry |
bool | false | Unknown. Maybe an unused duplicate of stop_this_entry ? When using snd_sos_show_operator_start 1, match_this_entry is listed, but stop_this_entry is not.
|
stop_oldest |
bool | true | Affects the choice of playing sounds if multiple are matching but not all are to be stopped. If set to true/false, stops oldest/youngest (the ones that were started the earliest/latest). |
invert_match |
bool | false | If set to true, stops all currently playing sounds except the matching sounds. |
Output Name | Type | Description |
---|---|---|
output_entries_matching |
float | The number of entries that matched the defined entry when this operator has finished execution.![]() "@your_stop_entry_operator_name.output_entries_matching" . |
output_this_matches_index |
float | Uncertain. It's an output so it'd be used in the same manner as output_entries_matching .
|
sys_start_entry
Plays another sound.
input_execute |
float | If its value is greater than 0.0 , this operation will execute. Default value is 1.0
|
input_start |
float | If its value is greater than 0.0 the sound entry will start. Default value is 1.0
|
entry_name |
string | The entry to play. |
input_start_delay |
float | Adds a delay before an instance is played. |
input_maintain_seed |
float | Greater than 0.0 will ensure that the rndwave selection is maintained.
|
sys_block_entries
Prevents new sounds from playing.
input_duration |
How long to suppress sounds for. | |
input_active |
![]() | |
match_entry |
Sound entry to suppress. | |
match_sound |
A raw WAV/MP3filename to match. Possibly deprecated? | |
match_substring |
Whether match_entry can be part of a larger string (e.g. "MyGun" matches MyGun.fire, MyGun.reload).
| |
match_channel |
Sound must have the same channel (e.g. CHAN_STATIC) as this one to match. | |
match_entity |
Sound must be coming from the same entity as this one. |
sys_output
Sends a value back to the sound system relating to the current entry.
input_float |
Takes a float value to send to the system. | |||||||||||||||||||
input_vec3 |
Vector. | |||||||||||||||||||
input_speakers |
Takes an array of floats to send to the system, each to do with a certain speaker. Use only with the speakers output. | |||||||||||||||||||
output |
string | Says what value should be altered. Legal values are:
|
sys_platform
Tests what platform the engine is running on.
pc |
bool | Should the operator return true if running on this platform? |
x360 |
bool | |
ps3 |
bool |
sys_mixlayer
scripts\soundmixers.txt
Configures a mixlayer which allows you to modify properties (volume
, level
, dsp
) of individual mixgroups
within the active soundmixer
. Mixlayers can be found and created in scripts\soundmixers.txt
.
mixlayer |
string | The layer to alter. |
mixgroup |
string | The sound group within the layer to alter. |
field |
string | Property of the group to alter. |
input |
float |
Maths
Generate or process numbers.
The result of these is stored in @name.output
.
math_random
Produces a random number.
input_min |
float | The lower bound for the random number. |
input_max |
float | The upper bound for the random number. |
round_to_int |
bool | Set to "true" or "false" (default). This makes the output a whole number.
|
math_float
Performs elementary maths operations with two values.
input1 |
float | Operand A. | ||||||||||||||||||||||||||||||||||
input2 |
float | Operand B. | ||||||||||||||||||||||||||||||||||
apply |
string | Specifies the operation to perform:
|
math_float_accumulate12
Does the same as math_float
, but uses 12 inputs instead. All examples use mult
.
|
float | Operands 1-12. |
apply |
string | The operation to perform, see #math_float. For mult the output is input1 * input2 * ... * input12 .
|
math_func1
Executes an unary math function.
input1 |
float | The argument to pass. | ||||||||||||||||||||||||
function |
string | Specifies the function to call:
| ||||||||||||||||||||||||
normalize_trig |
bool | If set to "true" , rescales the output from (−1, +1) to (0, 1).
|
math_logic_switch
Selects one of two possible outputs.
input_switch |
float | Chooses which of the other values is outputted. |
input1 |
float | The value to output if input_switch is 0 .
|
input2 |
float | The value to output if input_switch is 1 .
|
math_speakers
Performs math operations with an array of (6?) volumes.
To use a single float value as an input array consisting only of that value, do input2[*] 1.0
(which means input2 = [1,1,...,1]
).
This operator has the output named output
which can serve as an input for the input_speakers
attribute of #sys_output.
The operator #calc_spatialize_speakers has the output named output
which can serve as an input for this operator.
input1 |
float array | [1,1,...,1] | |||||
input2 |
float array | [1,1,...,1] | |||||
apply |
string | The arithmetic operation to perform.
| |||||
left_front right_front center lfe left_rear right_rear |
float | 1.0 | Another input value for a specific volume of the array. Note that these values don't need to be specified if the operation is mult and max since the default value of 1.0 results in a constant change in both cases. ![]() update_dialog_spatial (operator "speakers_limit", second to last) is weird. |
math_curve_2d_4knot
Multinode Remapper. Found in sound_operator_stacks.txt of Insurgency (2014) and
Day of Infamy.
curve_type
step
,linear
input
- Value that is compared to X: if input = X1 then ouput = Y1, if input = X2 then ouput = Y2, etc.
input_X1
input_Y1
input_X2
input_Y2
input_X3
input_Y3
input_X4
input_Y4
math_remap_float
- Remaps a value from one range to another (identically to math_remap).
input
<float>- The value to remap.
input_min
<float>input_max
<float>- The smallest/largest value the input will be set to.
input_map_min
<float>- When the input equals
input_min
, the output will be this value. input_map_max
<float>- When the input equals
input_max
, the output will be this value. default_to_max
<bool>clamp_range
<bool>
math_delta
input
<float>
Getters
Provide values from the engine.
get_convar
- Retrieve the value of a console variable.
convar
- The name of the console variable to read.
get_dashboard
get_map_name
- Checks if the given
map_name
matches the name of the map.
get_sys_time
- Collects the host/client time.
output_client_time
output_host_time
get_entry_time
- Collects information about the playing sound.
entry
- The entry to collect the information about
output_entry_elapsed
- The time this sound has been playing for.

output_sound_elapsed
output_stop_elapsed
- -1 if playing, otherwise the time spent after being stopped.
output_sound_duration
- The total length of the sound file this stack is playing.
- Example operator stack to sync one track to another (including looping tracks), needs to be put in start_stacks in sound_operator_stacks.txt!
"synchronize_track" {
"catch_entry" { // Used to catch the entry we're syncing to
"operator" "get_entry_time"
}
"sound_offset" { // We have to account for the multiple loops that can happen
"operator" "math_float"
"apply" "mod"
"input1" "@catch_entry.output_entry_elapsed" // Get the duration this sound has been playing for
"input2" "@catch_entry.output_sound_duration" // % it by the total duration of the file we're playing from
}
"invert_offset" { // We have to skip the duration, the delay paramater accepts negative values to do that
"operator" "math_float"
"apply" "mult"
"input1" "-1"
"input2" "@sound_offset.output"
}
"whoosh" { // Skip the seconds to sync
"operator" "sys_output"
"output" "delay"
"input_float" "@invert_offset.output"
}
//"printvar1" { // Optional to print the offset to the console that we have calculated
// "operator" "util_print_float"
// "input" "@invert_offset.output"
//}
}
- Example usage in a soundscript:
"my.music.basetrack" {...} // A soundscript that is running in the background that we'll be syncing TO
"my.music.overlay" { // We will be syncing this track to the upper one
// Of course you will have standard soundscript settings here
soundentry_version 2
operator_stacks {
start_stack {
import_stack synchronize_track
catch_entry {
entry "my.music.basetrack" // You have to set the name of the entry you're trying to synchronize to here
}
}
}
get_source_info
- Collects a variety of information about the sound source.
- Below are the data points output by this operator: @my_get_source_info_operator.output_position, etc..
output_entity_index
- Returns the index of the source entity.
output_position
- The position of the entity.
output_angles
- The rotation of the entity.
output_radius
- Only valid for the emitter.
output_volume
output_level
output_pitch
- The relevant values of the soundscript.
output_source_count
- The number of sounds (including this one) produced by the entity.
game_view_info
- Collects a variety of game-specific information about the player for specialised uses.
input_source_index
<float>output_position
<vector3>output_position_x
<float>output_position_y
<float>output_position_z
<float>output_angles
<vector3>output_velocity_vector
<vector3>output_velocity_vector_x
<float>output_velocity_vector_y
<float>output_velocity_vector_z
<float>output_velocity
<float>output_velocity_xy
<float>output_stop_elapsed
game_entity_info
- Collects a variety of game-specific information about an entity for specialised uses.
input_entity_index
<float>output_position
<vector3>output_position_x
<float>output_position_y
<float>output_position_z
<float>output_angles
<vector3>output_velocity_vector
<vector3>output_velocity_vector_x
<float>output_velocity_vector_y
<float>output_velocity_vector_z
<float>output_velocity
<float>output_velocity_xy
<float>
get_track_syncpoint
- Used to sync one sound entry to another.
- Example
get_track_syncpoint
"start_delay_to_track_sync_point"
{
// the start sync point for the file we are syncing to
"this_entry_syncpoints"
{
"operator" "get_track_syncpoint"
"syncpoint_list" "syncpoints_1"
"this_entry_syncpoints" "true"
}
"sync_track_syncpoints"
{
"operator" "get_track_syncpoint"
"syncpoint_list" "syncpoints_1"
"input_min_time_to_next_sync" "@this_entry_syncpoints.output_first_syncpoint"
"input_max_time_to_next_sync" "1000"
"match_entry" "DOTAMusic.BattleMusic"
}
// output our resulting delay value
"delay_output"
{
"operator" "sys_output"
"input_float" "@sync_track_syncpoints.output_time_to_next_syncpoint"
"output" "delay"
}
}
get_soundmixer
get_opvar_float
- Gets the value of an
opvar
created with theset_opvar_float
operator.opvar
<string>
Setters
Change values in the engine.
set_convar
set_opvar_float
increment_opvar_float
Calculations
Calculate pre-set algorithms.
calc_source_distance
- Calculates the distance from this sound source (
emitter
/entity
) to the listener.
calc_falloff
- Calculates the falloff of a sound based on the distance from the sound source (
emitter
/entity
) to the listener and the multiplied output of the sound entriessoundlevel
and thelevel
property value of its assignedmixgroup
within the activesoundmixer
.input_distance
- Sound source position. Generally the output of a
calc_source_distance
operation. input_level
- Sound source's level/attenuation. Generally the output of a multiplication operation of the sound entries
soundlevel
and themixgroup
level
within the activesoundmixer
.
calc_distant_dsp
- Same setup as
calc_falloff
. Scales thedsp
level defined in the sound's assignedmixgroup
within the activesoundmixer
based on distance.input_distance
- Sound source position. Generally the output of a
calc_source_distance
operation. input_level
- Sound source's level/attenuation. Generally the output of a multiplication operation of the sound entries
soundlevel
and themixgroup
level
within the activesoundmixer
. Note:The output of this operation is then multipled by the
dsp
property value (@get_soundmixer_op.output_dsp
) of the assignedmixgroup
within the activesoundmixer
, with the output then being input to asys_output
dsp
operation.
calc_spatialize_speakers
Calculates speaker volumes for a spatial sound, i. e. a sound that is emitted from some coordinates in the world as opposed to a sound that "plays everywhere". This operator has an output named output
which is an array of float volumes suitable as an input for #math_speakers.
input_position |
Vector | The location where the sound emitted from. |
input_radius |
float | The radius of a sphere around the input position. If the listener's distance to the sound source is...
|
input_distance |
float | The distance from the input_position at which the listener currently is.
|
input_rear_stereo_scale |
float | A factor to multiply the rear volumes with.[confirm] |
input_radius_min input_radius_max |
float | Seen in CS:GO (e. g. update_default ) where all occurrences are set to the two values 23 and 24, and never combined with input_radius .
|
calc_occlusion
- Performs audio occlusion calculations.
input_trace_interval
input_scalar
input_position
- Generally the output (
@get_info_op.output_position
) of aget_source_info
operation.
calc_angles_facing
- input_angles
- Generally the output (
@get_info_op.output_angles
) of aget_source_info
operation.
Utilities
util_print_float
- Displays a float in the console, along with the name of this operator.
input
- The floating point value to show.
util_pos_vec8
input_index
<float>input_entry_count
<float>- Output the corresponding input position: a value of
1.0
outputinput_position_1
. input_position_0
<vector3>input_position_1
<vector3>input_position_2
<vector3>input_position_3
<vector3>input_position_4
<vector3>input_position_5
<vector3>input_position_6
<vector3>input_position_7
<vector3>output_position
<vector3>output_max_index
<float>
Other
track_queue
prestart_stack
syncpoint_list
<string>
Example:This code is in the
operator_stacks
code block within the Sound Entry of the containing sound script, not insound_operator_stacks.txt
"soundentry_operator_data" { "track_data" { "start_point" "0.353" "end_point" "38" "track_name" "main" "priority" "1" "priority_override" "true" "syncpoints" { "syncpoints_1" { "1" "2.533" "2" "5.066" "3" "7.599" "4" "10.132" "5" "12.665" "6" "15.198" "7" "17.731" "8" "20.264" "9" "22.797" "10" "25.33" "11" "27.863" "12" "30.396" "13" "32.929" "14" "35.462" "15" "38" } } } } "prestart_stack" { "sync_track_syncpoints" { "operator" "track_queue" "syncpoint_list" "syncpoints_1" } }
track_update
autoqueue_entry_at_end_point
<string>- Sound Entry to auto queue.
track_stop
[Todo]
op_accumulate_ss_float
input
iterate_operator
Note:All four occurrences in sound_operator_stacks.txt/Portal 2 are commented out:
// this calculates the loudest volume of the ss clients // NOT NEEDED ALL SS OPS SELF-CONTAINGED // "volume_acc_ss_max" // { // "operator" "op_accumulate_ss_float" // "input" "volume_apply_occlusion.output" // "iterate_operator" "occlusion" // }
iterate_merge_speakers
input_max_iterations
iterate_operator
input