Use
(Redirected from +use)
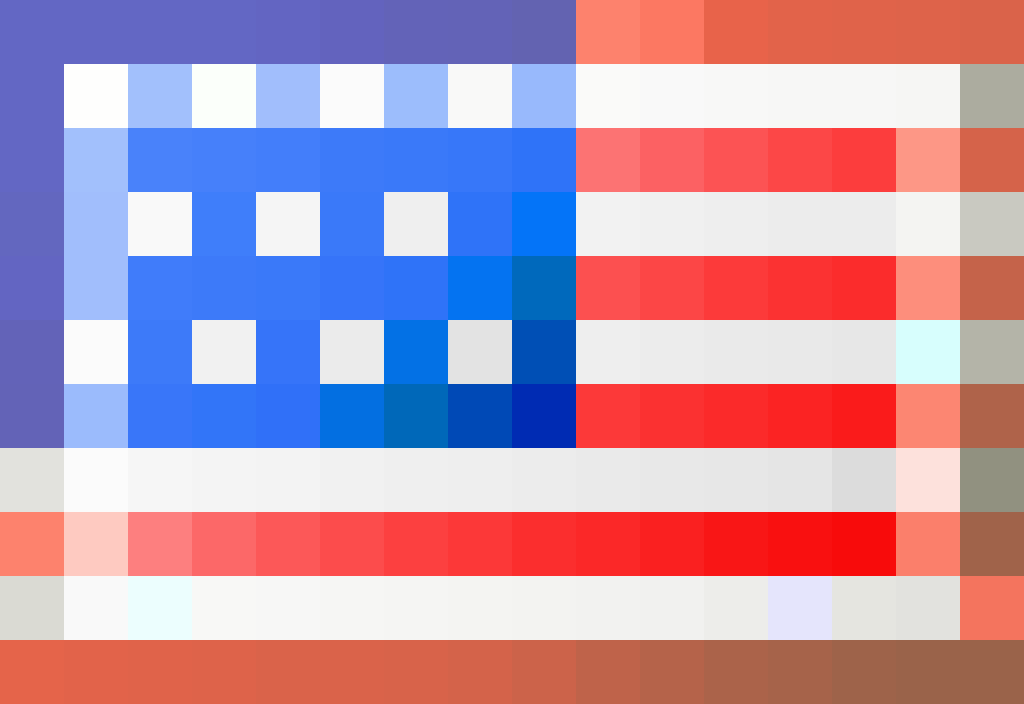

All entities have a Use input, though many do nothing in response to it. Typically, it performs the action players most expect when interacting with the entity (e.g., opening/closing a door, picking up a physics object). There are four methods to invoke it:
- A player looking at the entity and using the
+use
console command (commonly bound to the E key). - An entity output that specifies the
Use
input, or not specifying any input. - Directly calling the C++ function.
- If an entity without a use function is parented to one which does, it will passthrough the input to the parent.
Programming
Although there is always a Use
input, the actual Use
function of an entity starts null. It can be assigned at any time with SetUse( void *SomeFunc(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) )
.
It doesn't appear possible to specify USE_TYPE
or value
through the I/O system.