ai_goal_actbusy_queue
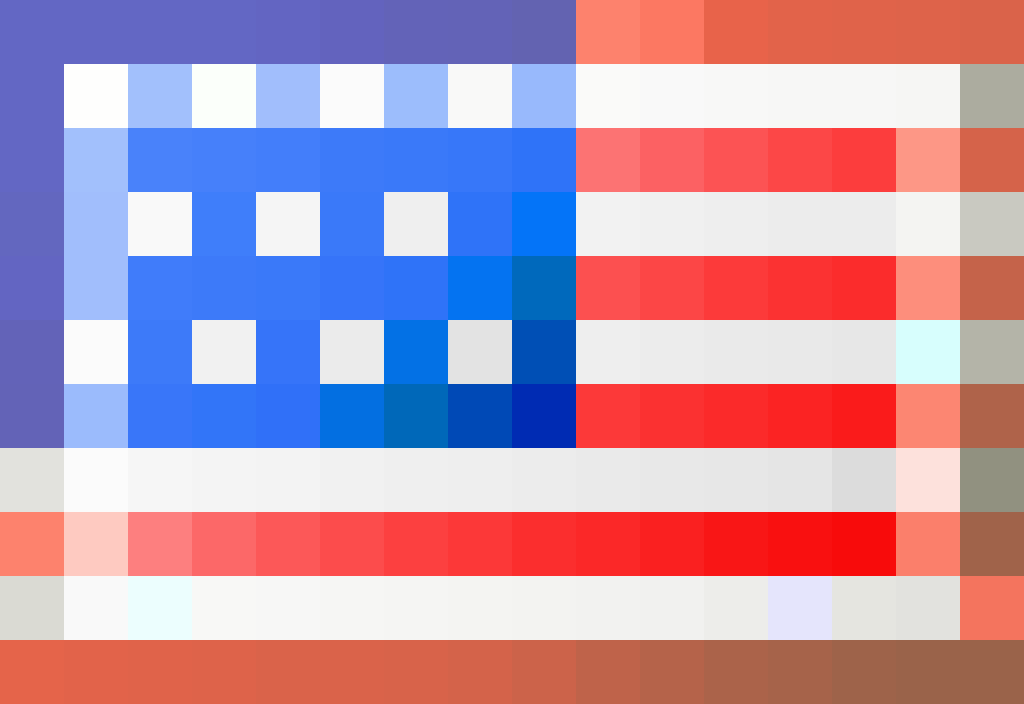

![]() |
---|
CAI_ActBusyQueueGoal |
![]() ai_behavior_actbusy.cpp
|
ai_goal_actbusy_queue
is a point entity available in Half-Life 2 and
Alien Swarm.
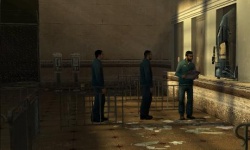
d1_trainstation_02
.It can do anything that an ai_goal_actbusy
can do, except this entity is designed to order actbusy hints and NPCs in a queue. The queue can be moved forward, and when the first NPC in the queue leaves, it can be given orders.


You can't just use a small delay there, cause it still interrupts extra other npc from queue and npc, who finished his busy activity react immediately, causing whole queue to stuck by his movements, cause he didn't proceed to exit node, also, you can't use OnNpcLeft and OnNpcLeftQueue (it just won't work in this case).

OnNPCFinishedBusy !activator AddOutput OnUser1 busy_relay:Trigger
OnNPCFinishedBusy !activator FireUser1 <delay:0.20>
In your logic_relay (busy_relay) you can set a delay to wait for npc before switching to other ai_goal_actbusy system like this:
OnTrigger actbusy_street_new ForceNPCToActBusy !activator <delay>
Contents
Keyvalues
- Name
(targetname)
<string> - The name that other entities refer to this entity by, via Inputs/Outputs or other keyvalues (e.g.
parentname
ortarget
).
Also displayed in Hammer's 2D views and Entity Report.See also: Generic Keyvalues, Inputs and Outputs available to all entities
- Exit Node
(node_exit)
<targetname> - The name of the first node (
info_node_hint
orpath_corner
) the first NPC in the queue should head to when leaving the queue. Required. - Node 1
(node1)
to Node 20 <targetname> - Used for defining the path of the queue, with
info_node_hint
s. Node 1 is the front of the queue. The number of defined nodes defines the maximum number of NPCs queuing. - Must Reach Front
(mustreachfront)
<choices> - If true, an NPC must strictly be at Node 1 before being able to leave the queue.
- 0: No
- 1: Yes
AI_ActBusyGoal:
- Search Range for Busy Hints
(busysearchrange)
<float> - Maximum distance between an actbusy hint and NPC for the NPC to consider it.
- Visible Busy Hints Only
(visibleonly)
<boolean> - If set, an NPC will only consider actbusy hints in view when deciding which to use. Once the choice has been made it will not change, even if new hints become visible.
- Actbusy Type
(type)
<choices> - Is this Actbusy part of combat? For use with Combat Safe Zone.
- 0: Default (Standard)
- 1: Combat
- Allow actor to teleport?
(allowteleport)
<boolean> - Sight Entity
(seeentity)
<targetname> - Optionally, if the Actor playing the ActBusy loses sight of this specified entity for an amount of time defined by Sight Entity Timeout, the specified entity will leave the ActBusy.
Note:Only targetnames are allowed, not classnames!
- Sight Entity Timeout
(seeentitytimeout)
<float> - Time in seconds to wait for an Actor to see the Sight Entity again before the entity may leave the ActBusy.
- Sight Enemy Method
(sightmethod)
<choices> - How to determine if the Actor sees the Sight Entity.
- Combat Safe Zone
(safezone)
<targetname> - Specify a brush entity to act as a safe zone if Actbusy Type is set to Combat. If any enemies are in the safe zone, the actbusy will break. Todo: Will actors go back to the actbusy once enemies are dead? What if they leave the safe zone but are still alive?
AI_GoalEntity:
- Actor(s) to affect
(actor)
<target_name_or_class> - The targetname or classname of any NPCs that will be included in this goal. Wildcards are supported.
- Search Type
(SearchType)
<choices> - What the Actor(s) to affect keyvalue targets by.
- 0: Entity Name
- 1: Classname
- Start Active
(StartActive)
<boolean> - Set if goal should be active immediately.
Inputs
MoveQueueUp
- Moves all NPCs in the queue up one position, and sends the first NPC to the Exit Node.
PlayerStartedBlocking
<float>- Tell the queue manager that the player has started blocking a specified point in the queue.
PlayerStoppedBlocking
<float>- Tell the queue manager that the player has stopped blocking a specified point in the queue.
AI_ActBusyGoal:
ForceNPCToActBusy
<string>- Format:
<targetname> [hint node targetname] [teleport] [nearest] [$<custom activity or sequence>] [max time]
- Force an NPC to act busy.
- The targetname parameter specifies the name of the NPC(s).
- The hint node targetname parameter specifies the name of the Hint node. Used to force an NPC to act busy on a specific node. If no hint node targetname is specified, it'll search for a random one.
- If the teleport keyword is specified as a parameter, the NPC will teleport onto the actbusy node instead of navigating to it.
- If the nearest keyword is specified as a parameter, the NPC will choose the nearest valid actbusy node, instead of choosing one randomly from all valid actbusy nodes.
- The max time parameter specifies the maximum time to actbusy. If no max time is specified, it'll use the default. Specifying 0 as the max time will make the NPC(s) act busy until disturbed.
- The $customactivityorsequence parameter specifies the name of a custom activity, prepended by a "$", that the NPC(s) will preform while navigating to the node, e.g.
$ACT_RUN
will make the NPC(s) run to the node. Sequence names can be used instead of activities.
ForceThisNPCToActBusy
<targetname>- Force an specified NPC to act busy. If there are multiple NPCs with the same name, only one will be ordered.
ForceThisNPCToLeave
<targetname>- Force an NPC to find an NPC exit point (
HINT_NPC_EXIT_POINT
hintnode) and vanish. Supports wildcards.
SetBusySearchRange
<float>- Alters the Search Range for Busy Hints keyvalue for all actors.
AI_GoalEntity:
Activate
- Make the goal active if it wasn't already. NPCs will begin taking orders.
Deactivate
- Make goal inactive.
UpdateActors
!FGD- Tell the goal entity to re-check Actor(s) to affect in case any disappeared, or any new NPCs spawned.[confirm]
Outputs
OnQueueMoved
<integer>- Fired when the queue moves up. This output automatically puts the number of NPCs remaining in the queue into the parameter box for inputs, if the mapper does not override the parameter with something else.
OnNPCLeftQueue
<targetname>- Fired when an NPC goes to the Exit Node. This output automatically puts the targetname of the NPC into the parameter box for inputs, if the mapper does not override the parameter with something else.
OnNPCStartedLeavingQueue
<targetname>- Fired when an NPC is at Node 1. This output automatically puts the targetname of the NPC into the parameter box for inputs, if the mapper does not override the parameter with something else.
AI_ActBusyGoal:
OnNPCStartedBusy
<targetname>- Fired when an NPC targeted by this entity starts an ActBusy animation sequence. This output automatically puts the targetname of the NPC into the parameter box for inputs, if the mapper does not override the parameter with something else.
OnNPCFinishedBusy
<targetname>- Fired when an NPC targeted by this entity finishes an ActBusy animation sequence. This output automatically puts the targetname of the NPC into the parameter box for inputs, if the mapper does not override the parameter with something else.
OnNPCLeft
<targetname>- Fired when an NPC targeted by this goal, ordered by the
ForceThisNPCToLeave
input, finishes its forced leave. This output automatically puts the targetname of the NPC into the parameter box for inputs, if the mapper does not override the parameter with something else.
OnNPCLostSeeEntity
<targetname>- Fired when the NPC loses sight of the Sight Entity. This output automatically puts the targetname of the NPC into the parameter box for inputs, if the mapper does not override the parameter with something else.
OnNPCSeeEnemy
<targetname>- Fired when this NPC leaves their ActBusy because they saw an enemy. This output automatically puts the targetname of the NPC (that left the actbusy, not the enemy) into the parameter box for inputs, if the mapper does not override the parameter with something else.
See also
- Actbusy Queues - Description of the Actbusy queue system.