Panel
(Redirected from VGUI Panel)
Jump to navigation
Jump to search
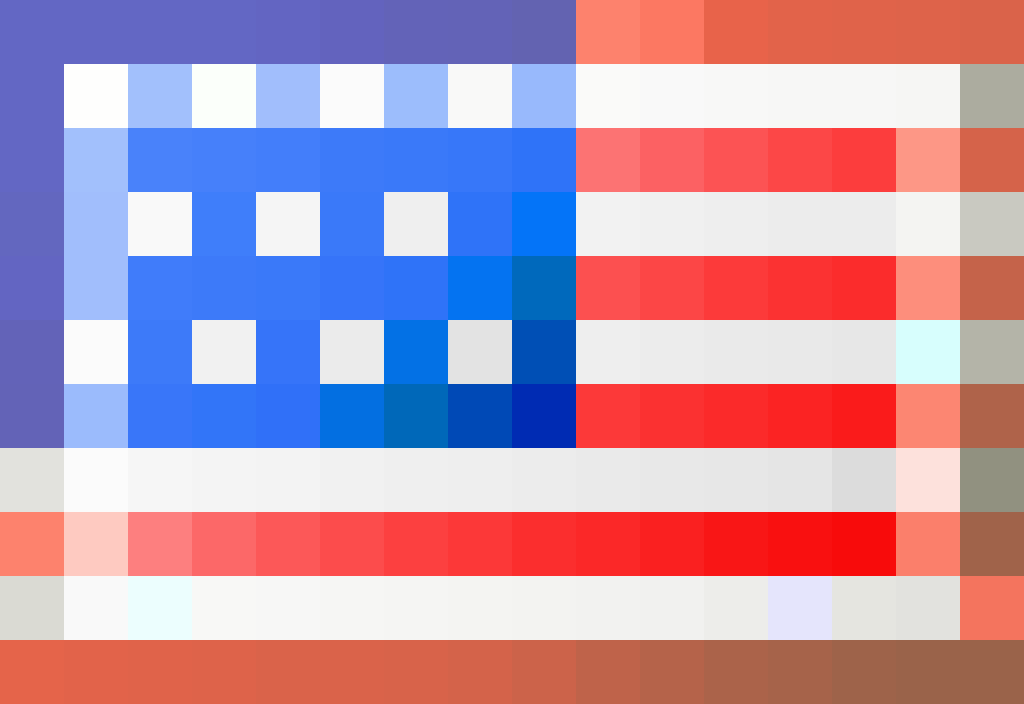

vgui::Panel
is the base class of all VGUI elements. It is a rectangle which covers an area of the screen and can draw itself, handle events, and host children.
Functions
General
SetVisible(bool)
bool IsVisible()
bool IsFullyVisible()
- Invisible panels and their children are not drawn or updated and do not receive input messages.
IsFullyVisible()
checks parent panels for visibility too. SetEnabled(bool)
IsEnabled()
- Disabled panels do not receive input and are often drawn with muted colours to indicate this.
OnThink()
- Called every frame before painting if the panel is visible. Todo: What about
Think()
? Is it obsolete? Does it run even if the panel isn't visible? void OnTick()
- Called at regular intervals if
ivgui()->AddTickSignal()
is called.
Layout
bool IsProportional()
SetProportional(bool)
Confirm:When a panel is proportional, its layout values are interpreted as being relative to a 640*480 screen. This allows you to position a panel at 64,48 and be certain that it will always be 10% away from the screen edge, regardless of the current resolution. This system does not respond well to changes in aspect ratio. The
c
andr
.res keywords were added to resolve this issue.SetPos(), GetPos(), SetSize(), GetSize(), SetBounds(), GetBounds(), GetWide(), SetWide(), GetTall(), SetTall()
- Functions for configuring the panel's position or size, or both at once.
Painting
A panel is drawn by its "paint" functions. PaintBackground()
and Paint()
are enabled by default.
PaintBackground()
SetPaintBackgroundEnabled(bool)
- Draws the background of the panel.
Paint()
SetPaintEnabled(bool)
- Draws the contents of the panel.
Confirm:Does not draw children.
PaintBorder()
SetPaintBorderEnabled(bool)
SetBorder(IBorder *border)
IBorder *GetBorder()
- Paints a border around the panel.
SetBorder()
must be called beforeSetPaintBorderEnabled()
.- Get an
IBorder
fromIScheme::GetBorder()
inApplySchemeSettings()
. - See
swarm/resource/sourcescheme.res
for examples of border definitions.
- Get an
PostChildPaint()
SetPostChildPaintEnabled(bool)
- Performs arbitrary drawing after the panel's children have been painted (i.e. above them).
SetSkipChildDuringPainting(Panel *child)
- Prevents a child from drawing, .
Confirm:without stopping it from thinking
SetAlpha(int)
- Sets the alpha of this panel and its children panels. 0 is invisible, 255 is opaque.
Hierarchy
Each panel has one parent and can have many children. Unless made a popup, children are drawn relative to and within the confines of their parent panel.
Parents
Panel *GetParent()
VPANEL GetVParent()
SetParent(Panel*)
orSetParent(VPANEL)
- Gets/sets this panel's parent.
bool HasParent(VPANEL)
- Is the given panel somewhere above this one in the hierarchy?
Children
int GetChildCount()
Panel *GetChild(int index)
Panel *FindChildByName(char* childName, bool recurseDown = false)
- Provides access to child panels.
OnChildAdded(VPANEL child)
- Called when a child is added to this panel.
Popups
PopupMakePopup(bool showTaskbarIcon = true,bool disabled = false)
bool IsPopup()
- Popup panels draw outside of their parent's space and do not follow it around.
Script Parameters
These can be used within a .res
layout file.
fieldName
<string>- Sets the control's name. Default is NULL.
wide
<integer>tall
<integer>- The initial size of the panel.
xpos
<integer>ypos
<integer>- The X and Y position of the panel's pinned corner relative to its parent. By default this value is an offset from the left/top edge, but the special characters
c
andr
allow positioning relative to the centre and right/bottom edge respectively. Examples:xpos 300
xpos r300
xpos c0
PinCorner
<choices>- The pinned corner is the one positioned at xpos/ypos. Values are:
TOPLEFT
(default)TOPRIGHT
BOTTOMLEFT
BOTTOMRIGHT
PinnedCornerOffsetX
<integer>PinnedCornerOffsetY
<integer>UnpinnedCornerOffsetX
<integer>UnpinnedCornerOffsetY
<integer>- [Todo]
zpos
<integer>- The Z position. Default is
1
. Higher numbers are drawn on top of lower numbers. IgnoreScheme
<boolean>- Todo: "Only get colors if we're ignoring the scheme."See also
IScheme
. visible
<boolean>- Whether the control is visible.
enabled
<boolean>- Whether the control can be used.
tabPosition
<integer>- Controls the order in which panels are focused on when Tab ⇆ is pressed. Valid range is 0-255. Default is 0.
tooltiptext
<string>- Text displayed when mouse is hovered over this panel. No support on consoles.
paintbackground
<boolean>paintborder
<boolean>- Whether to draw the background/border of the panel. Default is -1, which means no value will be applied.
AutoResize
<choices>- Sets which edges of the panel can be resized. Values are:
NO
(default)RIGHT
DOWN
DOWNANDRIGHT