Precaching assets
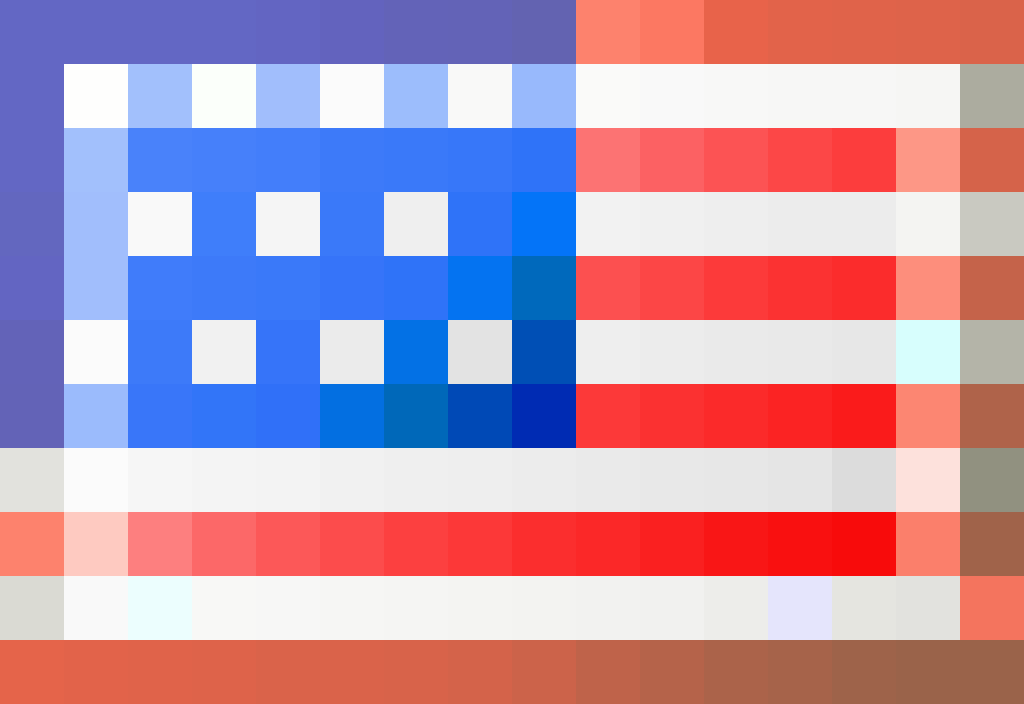

To provide a smooth loading of assets for a typical game session, it is important that the engine does as much work as possible to load those assets before the session begins. By doing this, the player's experience is not disturbed by interruptions as the engine tries to load assets in the middle of a session, often causing "hitching", lag or delays in rendering. With precaching, the assets are already loaded and are immediately ready for use. To do this, entities must declare and precache the assets they intend to use before the session starts. There are multiple utility functions in place to achieve this.
Functions
The Precache()
function is available to all descendants of the CBaseEntity
class and must be called in the Spawn()
function of each entity. All assets used by the entity (models, sounds, VCD, decals) must be declared in this function to be used. Assets may not be precached outside of this function.
Assets are precached using the functions below.
- PrecacheModel
- PrecacheMaterial
- PrecacheScriptSound
- PrecacheParticleSystem
- PrecacheStandardParticleSystem
- PrecacheGibsForModel
- PrecacheFileWeaponInfoDatabase
- PrecacheInstancedScene
- PrecachePhysicsSoundByStringIndex
- PrecachePhysicsSounds
- PrecachePointTemplates
- PrecacheVGuiScreen
- PrecacheVGuiScreenOverlayMaterial
- UTIL_PrecacheDecal
- UTIL_PrecacheOther
- UTIL_PrecacheOtherGrenade
PrecacheInstancedScene
Used to precache a VCD file.
void PrecacheInstancedScene( char const *pszScene )
Parameters
- pszScene
- Filename of the VCD to precache.
Return Value
- None.
UTIL_PrecacheOther
Utility function that will call the Precache()
function for an entity that will be referenced or created during the lifetime of the calling entity. This is often used for weapons that will create a child entity (like a grenade) dynamically during the course of a session.
void UTIL_PrecacheOther( const char *szClassname, const char *modelName )
Parameters
- szClassname
- Entity classname to precache assets for.
- modelName
- Model name to use for this entity.
Return Value
- None.
UTIL_PrecacheDecal
Used to precache a decal.
int UTIL_PrecacheDecal( const char *name, bool preload )
Parameters
- name
- Name of the decal to precache.
- preload
- Whether or not to preload this decal.
Return Value
- Reference index for the decal model.
More
- IEngineSound::PrecacheSound
- IVEngineServer::PrecacheSentenceFile
- IVEngineServer::PrecacheDecal
- IVEngineServer::PrecacheGeneric
Forcing Precache
If you have an entity you will be creating that isn't explicitly placed in the level, you can force precaching by modifying InitBodyQue as follows:
void InitBodyQue() { CBaseEntity *pEnt = CreateEntityByName("my_entity"); if ( pEnt ) { pEnt->Precache(); UTIL_Remove(pEnt); } }
Alternatively, certain entities like the player use the PRECACHE_REGISTER definition to force precache.
Defines
- PRECACHE_WEAPON_REGISTER
- CLIENTEFFECT_REGISTER_BEGIN