AllowWeaponSpawn
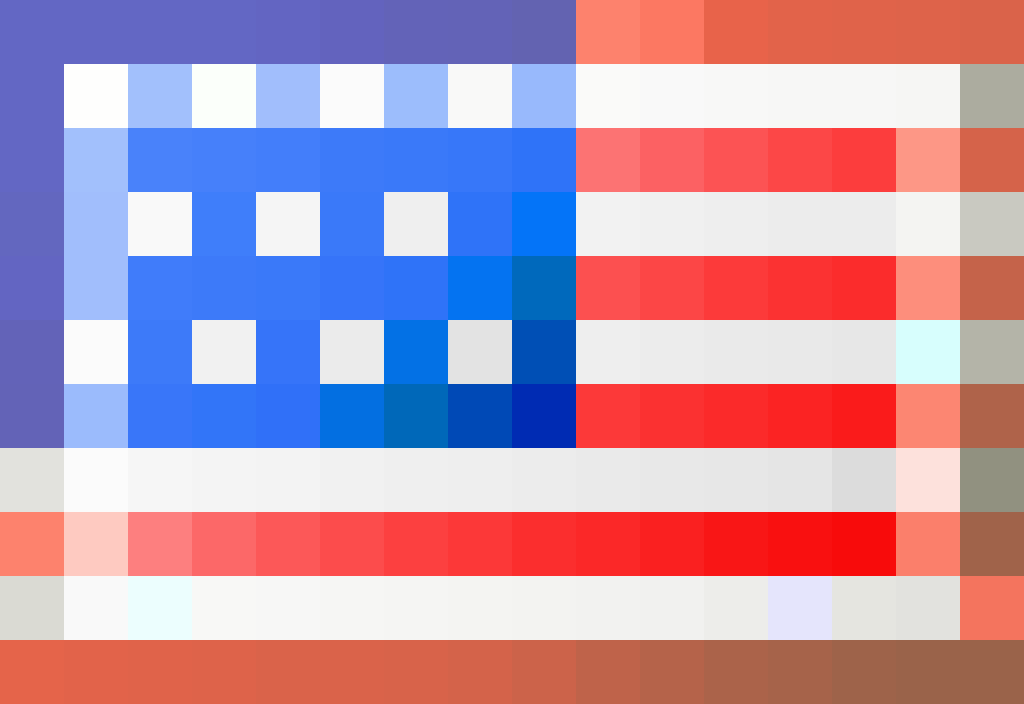

A callback for the DirectorOptions table. When DirectorOptions is loaded, this function will be called each time for all current weapons in the map, values being strings that represent their respective weapon entities, with a majority being classnames of the weapons's entities. Return true to allow the string's respective weapon to spawn.
Its best to see all the arguments value withprintl
first to know what to expect, especially since there are oddities for how weapon_ammo_spawn and upgrade_laser_sight are represented; Both respectively are represented asammo
andupgrade_item
strings. Like so:
Msg("VSCRIPT: Running director_base_addon.nut; VDC's!\n")
DirectorOptions <-
{
function AllowWeaponSpawn( classname )
{
printl(classname)
}
}
//----------------------------------------------
/*Should print something like:
** VSCRIPT: Running director_base_addon.nut; VDC's!
** weapon_rifle_ak47
** weapon_shotgun_spas
** weapon_rifle_desert
** weapon_sniper_military
** ammo
** weapon_chainsaw
** weapon_melee
** weapon_melee
** weapon_adrenaline
** upgrade_item
** [...]
*/
This callback can be undesirable to work with in certain occasions, as it can't account for specific melees, carryable props, or mounted guns. If so, refer to this sub-section of Code Samples section for an alternative.
Contents
Parameters
bool AllowWeaponSpawn(string classname)
Type | Name | Description |
---|---|---|
string | classname | Name of a weapon's classname, though a small few aren't their exact classnames. |
Expected Returns
Type | Description |
---|---|
bool | Return true to allow the respective weapon from spawning, false or none is otherwise. |
Code Samples
Array Approach
A common approach used in many mutations, including Valve's; This method makes the function find if the argument it gets called back is in theItemsToAllow
array, then it returns true if it finds it.
// Allow only rapid fire weapons originating in both L4D1 and CS:S, but also melees and ammo
DirectorOptions <-
{
ItemsToAllow =
[
"weapon_rifle"
"weapon_rifle_sg552"
"weapon_smg"
"weapon_smg_mp5"
"weapon_melee"
"ammo"
]
function AllowWeaponSpawn( classname )
{
// these zero byte strings are 'weapon_spawn' entities before they spawn a weapon
// always return true so the callback can get its weapon later
if( classname in ItemsToAllow || classname == "" )
return true
else
return false
}
}
String Similiarity Approach
In the example below, the function will only look for strings that contain a specific keyword in it. This is meant to be used if you need all weapons under a specific weapon category (shotguns, smgs) to be allowed, otherwise just use the above example, as you'll probably add unneeded complexity for your function.
DirectorOptions <-
{
TypesToAllow =
[
"rifle" // all rifles, T2
"shotgun" // all shotties, T1 + T2
]
function AllowWeaponSpawn( classname )
{
// these are 'weapon_spawn' entities before they spawn a weapon
// always return true so the callback can get its weapon later
if( classname == "" )
return false
foreach( weptype in TypesToAllow )
{
if( classname.find(weptype) )
{
printl(classname+"; Is: "+weptype)
return true
}
}
// Only with weapons that hasn't returned true in the 'foreach' loop will reach here
// So we can put a 'return false' here with no worries
return false
}
}
Custom 'AllowWeaponSpawn' Implementation
Sometimes, this callback may be undesirable to use, as it can't account for specific melees, carryable props, or mounted guns. Using OnGameEvent_' prefixed functions, hook the eventround_start_post_nav
and make our own 'AllowWeaponSpawn' implementation.
This implementation works by comparing the items's model path names, and deleting them if their model paths match the ones in theItemstoRemove_ModelPaths
array.
// Deletes weapons based on model name, meant for things like melee and carryables as 'AllowWeaponSpawn' DirectorOptions hook doesn't support doing so.
// You can use 'script __RunGameEventCallbacks("round_start_post_nav", {})' to force the game event callback to run after its registered
function OnGameEvent_round_start_post_nav( params )
{
// Keep our model path names here; Can be fetched with the .GetModelName() method for CBaseEntity
//// For melees, it doesn't remove ones already in a player's inventory, as those are "predicted_viewmodel" entities using the v_* prefix models.
local ItemstoRemove_ModelPaths =
[
// ** Melees **
"models/weapons/melee/w_bat.mdl", // Baseball Bat
"models/weapons/melee/w_cricket_bat.mdl",
"models/weapons/melee/w_crowbar.mdl",
"models/weapons/melee/w_electric_guitar.mdl",
"models/weapons/melee/w_fireaxe.mdl",
"models/weapons/melee/w_frying_pan.mdl",
"models/weapons/melee/w_golfclub.mdl",
"models/weapons/melee/w_katana.mdl",
"models/weapons/melee/w_machete.mdl",
"models/weapons/melee/w_pitchfork.mdl",
"models/weapons/melee/w_shovel.mdl",
"models/weapons/melee/w_tonfa.mdl",
"models/w_models/weapons/w_knife_t.mdl", // CS:S Knife
// ** Mounted Guns **
"models/w_models/weapons/w_minigun.mdl", // 'prop_minigun_l4d1'; Rapid firing gatling minigun found in maps like No Mercy
"models/w_models/weapons/50cal.mdl", // 'prop_mounted_machine_gun' / 'prop_minigun'; The minigun found in Swamp Fever - Plantation
// ** Carryable Props **
"models/props_junk/gascan001a.mdl",
"models/props_junk/explosive_box001.mdl", // Fireworks crate
"models/props_equipment/oxygentank01.mdl",
"models/props_junk/propanecanister001a.mdl",
]
// automatically grab each index in the array
foreach( modelpath in ItemstoRemove_ModelPaths )
{
local weapon_ent = null
while( weapon_ent = Entities.FindByModel(weapon_ent, modelpath) )
weapon_ent.Kill()
}
}