Game Window Cursor Clipping
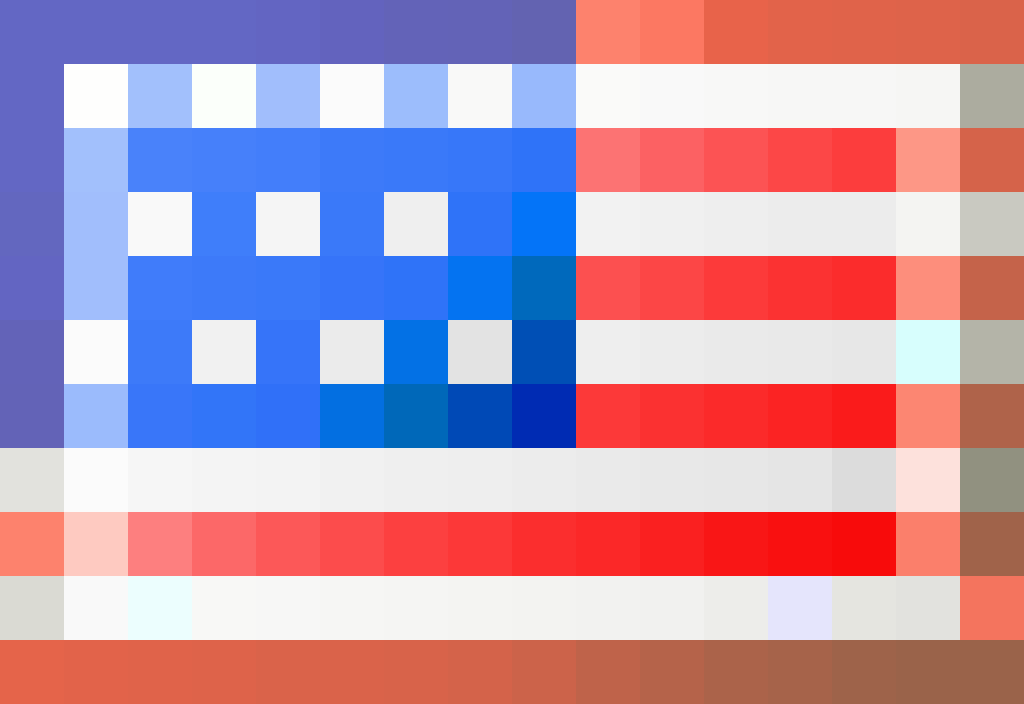

Summary
This implementation allows you to clip the cursor to the game window such as in DOOM and DOOM Eternal (id Tech 6 - 7), also brings some fixes to various bugs on VGUI. Those games are only meant to be examples and might not do it in the way we'll do below.



Requirements
- Knowledge of C++
- Knowledge of Source Engine
- Source SDK Base 2013 - Currently tested on Source SDK Base 2013 Multiplayer
Getting the Implementation/Code
First of all, you'll need the following files from the following GitHub repository:
Which you will copy to src/game/client/. You can also create a subfolder if you wish to, and copy these files there.
Implementation
Now head over to cdll_client_int.cpp and include
#include "CCursorClipManagement.h"
Anywhere before
// memdbgon must be the last include file in a .cpp file!!!
#include "tier0/memdbgon.h"

In the same cpp, find CHLClient::PostInit() and add this to the end:
CCursorClipManagement::Init();
Now find void OnRenderEnd() in the same cpp and add this to the end:
g_pCursorClipManager->Think();
Once you're done with the steps above, head over to baseviewport.cpp and add the following:
#include "../CCursorClipManagement.h"
After
// our definition
#include "baseviewport.h"
#include <filesystem.h>
#include <convar.h>
#include "ienginevgui.h"
#include "iclientmode.h"
Before
#include "tier0/etwprof.h"
Lastly, find CBaseViewport::OnScreenSizeChanged(int iOldWide, int iOldTall) and add this to the end:
g_pCursorClipManager->SetLockAction(CURSOR_CLIPMANAGEMENT_RELOCKREQUIRED);

Conclusion
You're all set! Compile your client project and check if it works.
Notes
- If you start the game with -tools, -dev, -developer, or -nocursorclipping, the cursor clipping will be disabled and won't work by default.
- If you're compiling into a different platform, else than WIN32, the cursor clipping will be disabled and won't work by default.
- You can also add a ConVar to enable or disable this dynamically in-game, all you'll need to do is adding one more check on CCursorClipManagement::Think().
- If you do not want to check whether the window is active or not every time OnRenderEnd() gets called, you can also do this by hooking into window events/messages. Check SetWindowsHookExA.