Flag
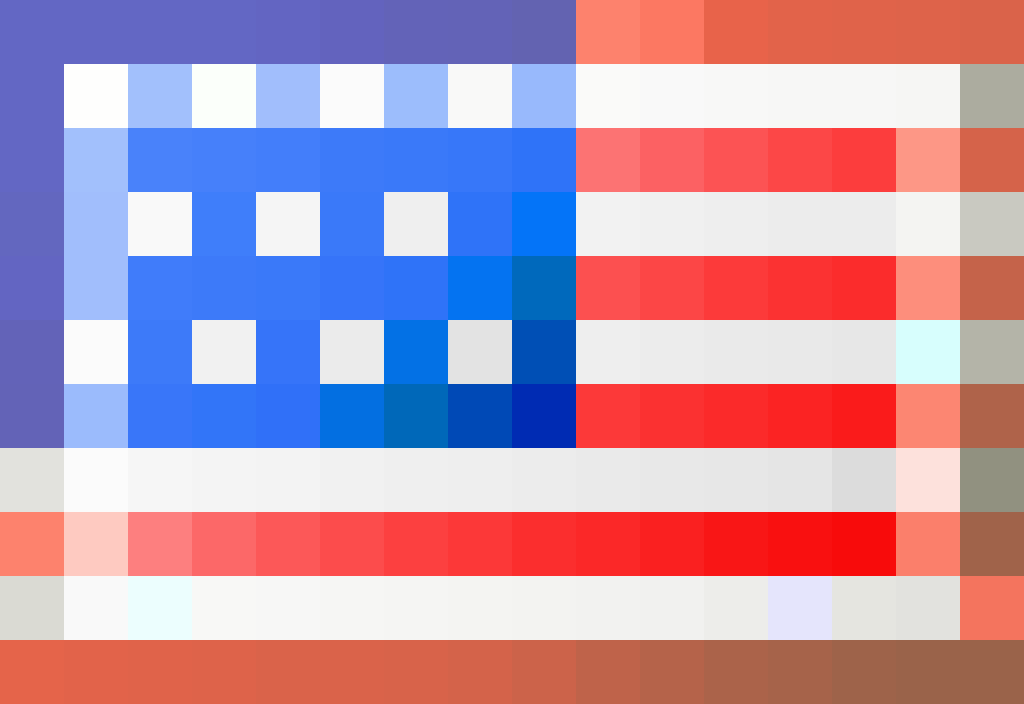

A Flag (bitmask or mask) is a boolean value that is stored within a large variable. This is advantageous because the smallest amount of memory that a program can allocate is 1 byte (or 8 bits), but a boolean only strictly needs 1 bit. If you have a lot of booleans to store, flags are eight times more efficient than the dedicated bool
type.
Entities in Source commonly use the following flag types:
- Spawn flags -
SF_
defined in each entity's.cpp
file - Behavior flags -
FL_
flags defined inpublic/const.h
- Effect flags -
EF_
flags defined inpublic/const.h
- Entity flags (aka
EFlags
) -EFL_
flags defined ingame/shared/shareddefs.h
Creation
Flags are typically defined as an enum like this:
enum MyFlags_t
{
FL_MYFLAG = 1 << 0,
FL_MYFLAG = 1 << 1,
FL_MYFLAG = 1 << 2,
// etc.
};
This is the same as:
enum MyFlags_t
{
FL_MYFLAG = 1,
FL_MYFLAG = 2,
FL_MYFLAG = 4,
// etc.
};
Older code uses the preprocessor to define flags, although this should be avoided as it is not strongly typed:
#define FL_MYFLAG 1<<0
#define FL_MYFLAG 1<<1
#define FL_MYFLAG 1<<2
// etc.
Usage
Flags should be managed through Source's dedicated functions, never directly. There are several different types of flags and each has its own set of functions, but they all follow the same naming pattern:
Add<*>Flags()
Remove<*>Flags()
Clear<*>Flags()
Has<*>Flags()
(sometimesIs<*>FlagSet()
)
These are the functions to call for common flag types:
Prefix | Add | Remove | Check | Clear | Get All | Internal Field | |
---|---|---|---|---|---|---|---|
Spawn Flags | SF_
|
AddSpawnFlags
|
RemoveSpawnFlags
|
HasSpawnFlags
|
ClearSpawnFlags
|
GetSpawnFlags
|
m_spawnflags
|
Behavior Flags | FL_
|
AddFlag
|
RemoveFlag
|
N/A | ClearFlags
|
GetFlags
|
m_fFlags
|
Effect Flags | EF_
|
AddEffects
|
RemoveEffects
|
IsEffectActive
|
ClearEffects
|
GetEffects
|
m_fEffects
|
EFlags | EFL_
|
AddEFlags
|
RemoveEFlags
|
IsEFlagSet
|
N/A | GetEFlags
|
m_iEFlags
|


EFL_
and EF_
, so always double check. Some of Valve's own code makes these mistakes!
To operate on several flags at once you must use the bitwise operators &
, |
and ^
- the same as the normal boolean operators, but with only one character.
For instance:
if ( HasSpawnFlags(SF_CITIZEN_MEDIC | SF_CITIZEN_NOT_COMMANDABLE) ) ...
Flags in Hammer
Flags appear in object properties in Valve Hammer Editor. Different objects will have different flags available. A flag appears as a checkbox, meaning each flag can be either on or off. You can also access flags as a keyvalue, spawnflags
. That's what the flags actually are; a specific number determined by the combination of flags that are checked.
